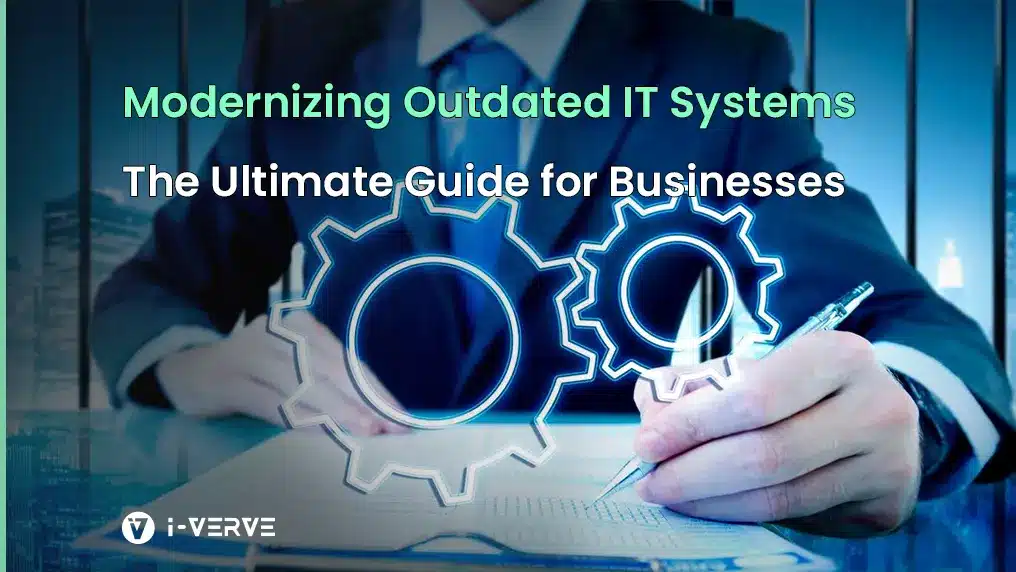
-
- Posted by Nayan
March 17, 2025
9 min read
In today’s fast-evolving technological world, mid-sized businesses often face a critical challenge...
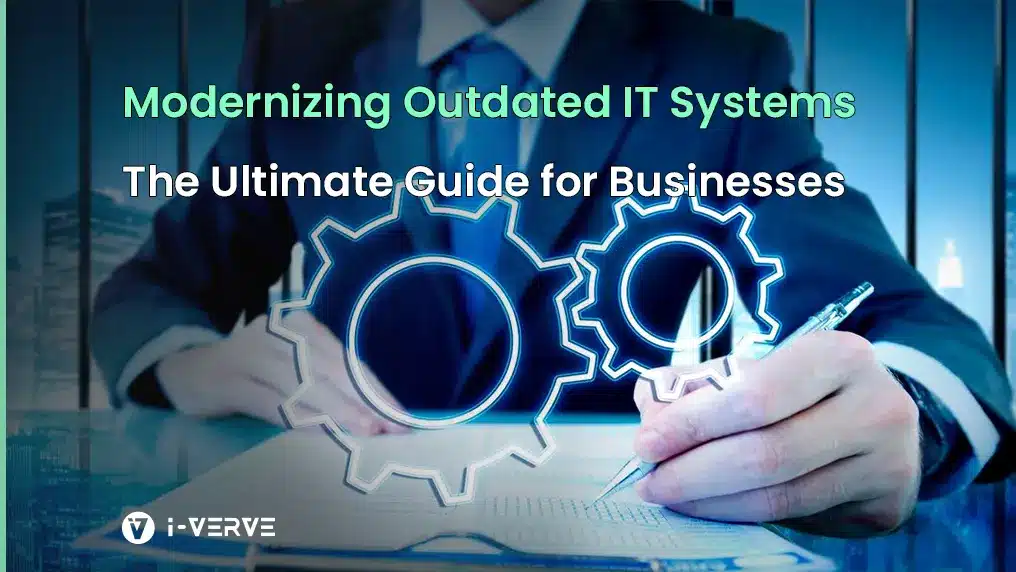
-
- Posted by Nayan
March 17, 2025
9 min read
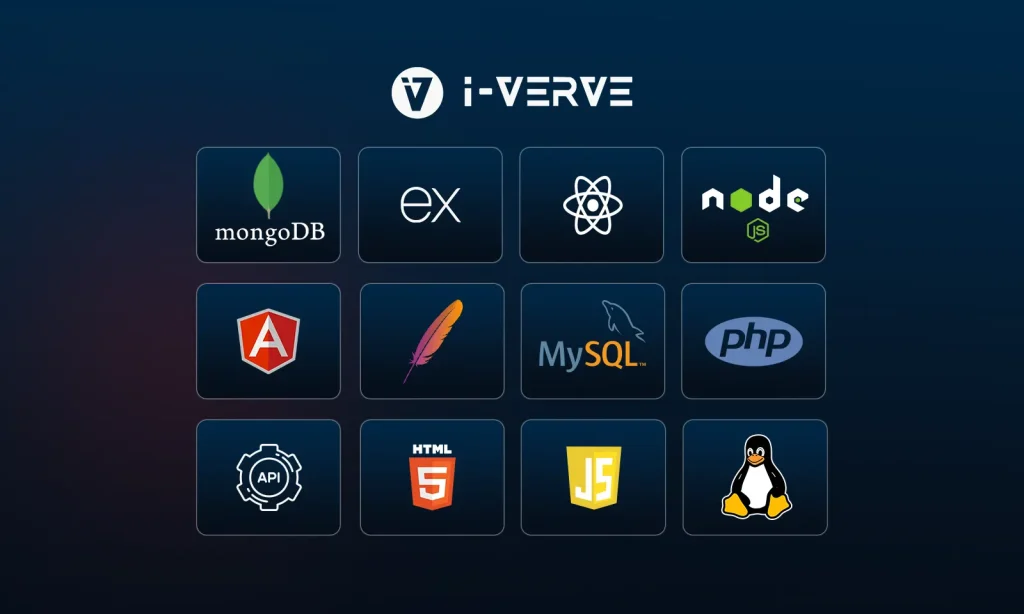
-
- Posted by Nayan
January 8, 2025
24 min read
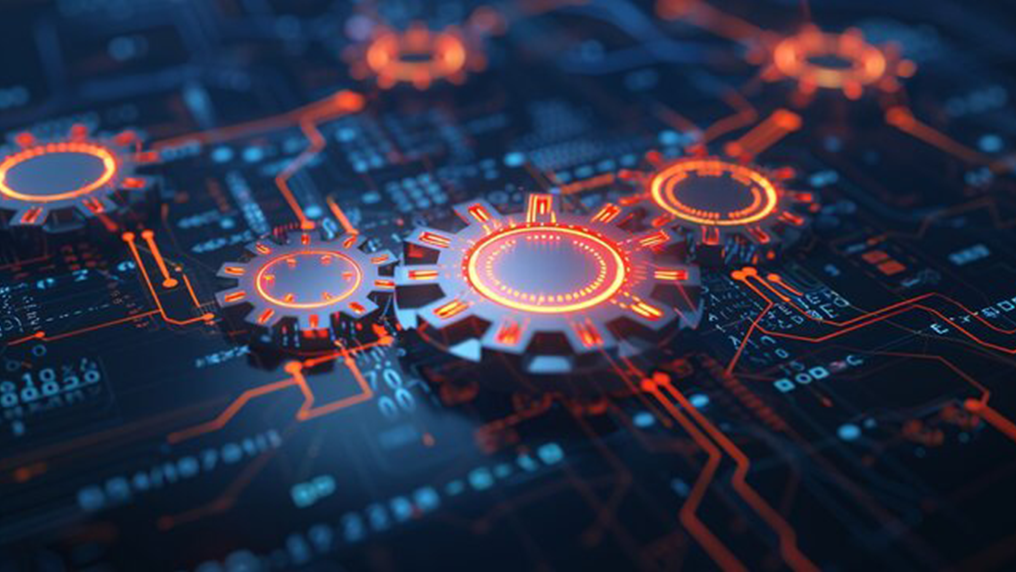
-
- Posted by Nayan
December 5, 2024
10 min read
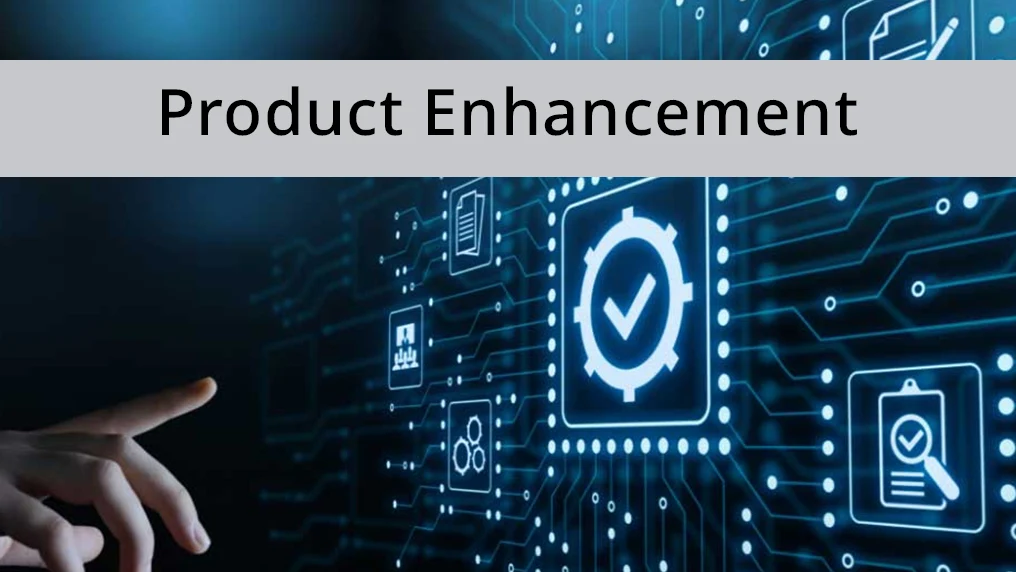
-
- Posted by Nayan
October 20, 2024
19 min read
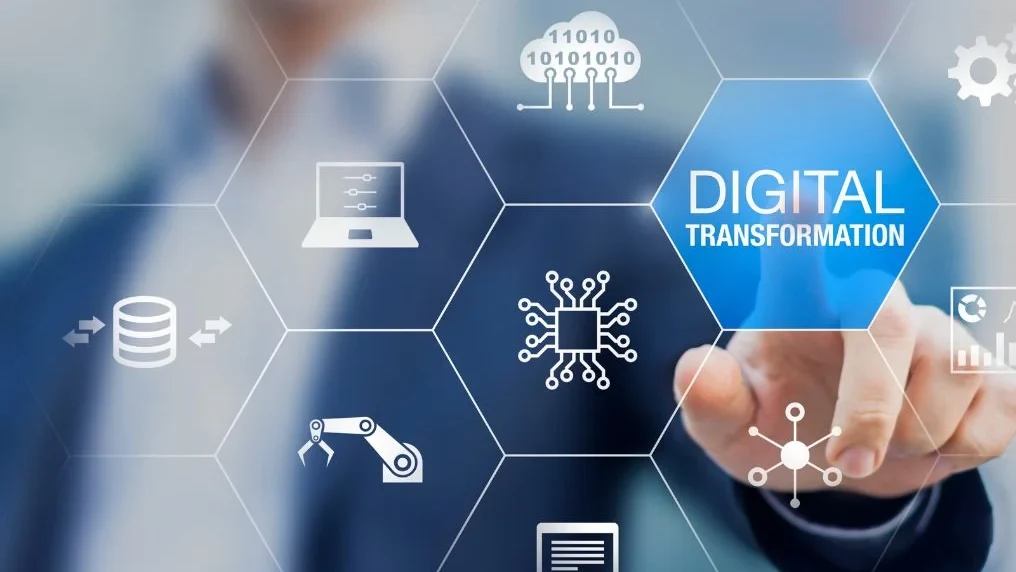
-
- Posted by Nayan
October 15, 2024
16 min read
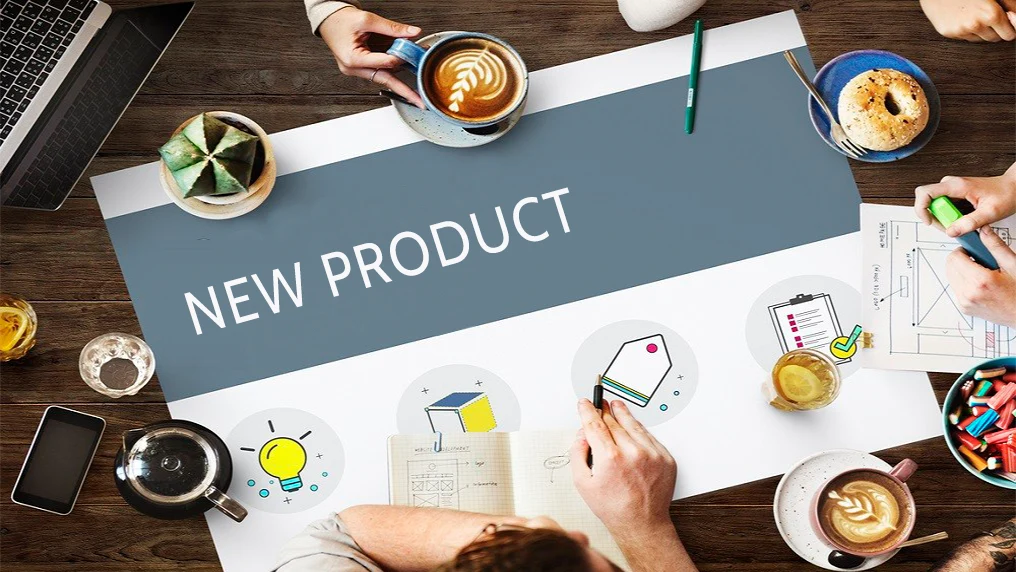
-
- Posted by Nayan
October 1, 2024
15 min read